ompl::geometric::eitstar::Vertex Class Reference
The vertex class for both the forward and reverse search. More...
#include <ompl/geometric/planners/informedtrees/eitstar/Vertex.h>
Inheritance diagram for ompl::geometric::eitstar::Vertex:
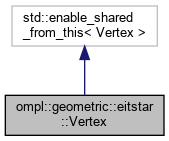
Public Member Functions | |
Vertex (const std::shared_ptr< State > &state, const std::shared_ptr< ompl::base::OptimizationObjective > &objective, const Direction &direction) | |
Constructs the vertex, which must be associated with a state. | |
~Vertex () | |
Destructs this vertex. | |
std::size_t | getId () const |
Gets the unique vertex-id of this vertex. | |
std::shared_ptr< State > | getState () const |
Returns the state associated with this vertex. | |
const std::vector< std::shared_ptr< Vertex > > & | getChildren () const |
Returns the children of this vertex. | |
bool | hasChildren () const |
Returns whether this vertex has children. | |
std::vector< std::shared_ptr< Vertex > > | updateCurrentCostOfChildren (const std::shared_ptr< ompl::base::OptimizationObjective > &objective) |
Update the cost-to-come of this vertex's children. | |
void | addChild (const std::shared_ptr< Vertex > &vertex) |
Adds the given vertex to this vertex's children. | |
void | removeChild (const std::shared_ptr< Vertex > &vertex) |
Removes the given vertex from this vertex's children. | |
std::weak_ptr< Vertex > | getParent () const |
Returns the parent of this vertex. | |
bool | isParent (const std::shared_ptr< Vertex > &vertex) const |
Returns whether the given vertex is this vertex's parent. | |
std::weak_ptr< Vertex > | getTwin () const |
Returns the twin of this vertex, i.e., the vertex in the other search tree with the same underlying state. | |
void | updateParent (const std::shared_ptr< Vertex > &vertex) |
Resets the parent of this vertex. | |
void | setEdgeCost (const ompl::base::Cost &edgeCost) |
Sets the cost of the edge in the forward tree that leads to this vertex. | |
ompl::base::Cost | getEdgeCost () const |
Returns the cost of the edge in the forward tree that leads to this vertex. | |
void | resetParent () |
Returns the parent of this vertex. | |
void | setTwin (const std::shared_ptr< Vertex > &vertex) |
Sets the twin of this vertex, i.e., the vertex in the other search tree with the same underlying state. | |
void | clearChildren () |
Resets the children of this vertex. | |
std::size_t | getExpandTag () const |
Returns the tag when this vertex was last expanded. | |
void | registerExpansionInReverseSearch (std::size_t tag) |
Sets the expand tag when this vertex was last expanded. | |
void | callOnBranch (const std::function< void(const std::shared_ptr< eitstar::State > &)> &function) |
Recursively calls the given function on this vertex and all its children in the tree. | |
Friends | |
class | ReverseQueue |
The edge queue is a friend class to allow efficient updates of outgoing edges of this vertex in the queue. | |
Detailed Description
The documentation for this class was generated from the following files:
- ompl/geometric/planners/informedtrees/eitstar/Vertex.h
- ompl/geometric/planners/informedtrees/eitstar/src/Vertex.cpp